mirror of
https://github.com/cwinfo/matterbridge.git
synced 2024-09-20 17:12:31 +00:00
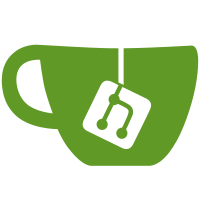
TengoModifyMessage allows you to specify the location of a tengo (https://github.com/d5/tengo/) script. This script will receive every incoming message and can be used to modify the Username and the Text of that message. The script will have the following global variables: to modify: msgUsername and msgText to read: msgChannel and msgAccount The script is reloaded on every message, so you can modify the script on the fly. Example script can be found in https://github.com/42wim/matterbridge/tree/master/gateway/bench.tengo and https://github.com/42wim/matterbridge/tree/master/contrib/example.tengo The example below will check if the text contains blah and if so, it'll replace the text and the username of that message. text := import("text") if text.re_match("blah",msgText) { msgText="replaced by this" msgUsername="fakeuser" } More information about tengo on: https://github.com/d5/tengo/blob/master/docs/tutorial.md and https://github.com/d5/tengo/blob/master/docs/stdlib.md
106 lines
2.0 KiB
Go
106 lines
2.0 KiB
Go
package objects
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
"github.com/d5/tengo/compiler/token"
|
|
)
|
|
|
|
// ImmutableMap represents an immutable map object.
|
|
type ImmutableMap struct {
|
|
Value map[string]Object
|
|
}
|
|
|
|
// TypeName returns the name of the type.
|
|
func (o *ImmutableMap) TypeName() string {
|
|
return "immutable-map"
|
|
}
|
|
|
|
func (o *ImmutableMap) String() string {
|
|
var pairs []string
|
|
for k, v := range o.Value {
|
|
pairs = append(pairs, fmt.Sprintf("%s: %s", k, v.String()))
|
|
}
|
|
|
|
return fmt.Sprintf("{%s}", strings.Join(pairs, ", "))
|
|
}
|
|
|
|
// BinaryOp returns another object that is the result of
|
|
// a given binary operator and a right-hand side object.
|
|
func (o *ImmutableMap) BinaryOp(op token.Token, rhs Object) (Object, error) {
|
|
return nil, ErrInvalidOperator
|
|
}
|
|
|
|
// Copy returns a copy of the type.
|
|
func (o *ImmutableMap) Copy() Object {
|
|
c := make(map[string]Object)
|
|
for k, v := range o.Value {
|
|
c[k] = v.Copy()
|
|
}
|
|
|
|
return &Map{Value: c}
|
|
}
|
|
|
|
// IsFalsy returns true if the value of the type is falsy.
|
|
func (o *ImmutableMap) IsFalsy() bool {
|
|
return len(o.Value) == 0
|
|
}
|
|
|
|
// IndexGet returns the value for the given key.
|
|
func (o *ImmutableMap) IndexGet(index Object) (res Object, err error) {
|
|
strIdx, ok := ToString(index)
|
|
if !ok {
|
|
err = ErrInvalidIndexType
|
|
return
|
|
}
|
|
|
|
val, ok := o.Value[strIdx]
|
|
if !ok {
|
|
val = UndefinedValue
|
|
}
|
|
|
|
return val, nil
|
|
}
|
|
|
|
// Equals returns true if the value of the type
|
|
// is equal to the value of another object.
|
|
func (o *ImmutableMap) Equals(x Object) bool {
|
|
var xVal map[string]Object
|
|
switch x := x.(type) {
|
|
case *Map:
|
|
xVal = x.Value
|
|
case *ImmutableMap:
|
|
xVal = x.Value
|
|
default:
|
|
return false
|
|
}
|
|
|
|
if len(o.Value) != len(xVal) {
|
|
return false
|
|
}
|
|
|
|
for k, v := range o.Value {
|
|
tv := xVal[k]
|
|
if !v.Equals(tv) {
|
|
return false
|
|
}
|
|
}
|
|
|
|
return true
|
|
}
|
|
|
|
// Iterate creates an immutable map iterator.
|
|
func (o *ImmutableMap) Iterate() Iterator {
|
|
var keys []string
|
|
for k := range o.Value {
|
|
keys = append(keys, k)
|
|
}
|
|
|
|
return &MapIterator{
|
|
v: o.Value,
|
|
k: keys,
|
|
l: len(keys),
|
|
}
|
|
}
|