mirror of
https://github.com/cwinfo/matterbridge.git
synced 2024-11-13 04:30:26 +00:00
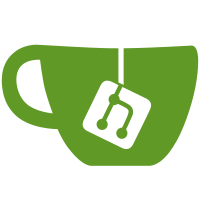
Harmony is a relatively new (1,5yo) chat protocol with a small community. This introduces support for Harmony into Matterbridge, using the functionality specifically designed for bridge bots. The implementation is a modest 200 lines of code.
2191 lines
73 KiB
Go
2191 lines
73 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.23.0
|
|
// protoc v3.17.3
|
|
// source: auth/v1/auth.proto
|
|
|
|
package authv1
|
|
|
|
import (
|
|
proto "github.com/golang/protobuf/proto"
|
|
v1 "github.com/harmony-development/shibshib/gen/harmonytypes/v1"
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
// This is a compile-time assertion that a sufficiently up-to-date version
|
|
// of the legacy proto package is being used.
|
|
const _ = proto.ProtoPackageIsVersion4
|
|
|
|
// Used in `BeginAuth` endpoint.
|
|
type BeginAuthRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *BeginAuthRequest) Reset() {
|
|
*x = BeginAuthRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BeginAuthRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BeginAuthRequest) ProtoMessage() {}
|
|
|
|
func (x *BeginAuthRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BeginAuthRequest.ProtoReflect.Descriptor instead.
|
|
func (*BeginAuthRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
// BeginAuthResponse
|
|
// The return type of BeginAuth, containing the
|
|
// auth_id that will be used for the authentication
|
|
// section
|
|
type BeginAuthResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// auth_id: the ID of this auth session
|
|
AuthId string `protobuf:"bytes,1,opt,name=auth_id,json=authId,proto3" json:"auth_id,omitempty"`
|
|
}
|
|
|
|
func (x *BeginAuthResponse) Reset() {
|
|
*x = BeginAuthResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BeginAuthResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BeginAuthResponse) ProtoMessage() {}
|
|
|
|
func (x *BeginAuthResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BeginAuthResponse.ProtoReflect.Descriptor instead.
|
|
func (*BeginAuthResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *BeginAuthResponse) GetAuthId() string {
|
|
if x != nil {
|
|
return x.AuthId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Session
|
|
// Session contains the information for a new session;
|
|
// the user_id you logged in as and the session_token
|
|
// which should be passed to authorisation
|
|
type Session struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// user_id: the ID of the user you logged in as
|
|
UserId uint64 `protobuf:"varint,1,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
// session_token: the session token to use in authorization
|
|
SessionToken string `protobuf:"bytes,2,opt,name=session_token,json=sessionToken,proto3" json:"session_token,omitempty"`
|
|
}
|
|
|
|
func (x *Session) Reset() {
|
|
*x = Session{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Session) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Session) ProtoMessage() {}
|
|
|
|
func (x *Session) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[2]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Session.ProtoReflect.Descriptor instead.
|
|
func (*Session) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *Session) GetUserId() uint64 {
|
|
if x != nil {
|
|
return x.UserId
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *Session) GetSessionToken() string {
|
|
if x != nil {
|
|
return x.SessionToken
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// AuthStep
|
|
// A step in the authentication process
|
|
// Contains a variety of different types of views
|
|
// It is recommended to have a fallback_url specified
|
|
// For non-trivial authentication procedures (such as captchas)
|
|
type AuthStep struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// fallback_url: unused
|
|
FallbackUrl string `protobuf:"bytes,1,opt,name=fallback_url,json=fallbackUrl,proto3" json:"fallback_url,omitempty"`
|
|
// can_go_back: whether or not the client can request the
|
|
// server to send the previous step
|
|
CanGoBack bool `protobuf:"varint,2,opt,name=can_go_back,json=canGoBack,proto3" json:"can_go_back,omitempty"`
|
|
// step: the current step
|
|
//
|
|
// Types that are assignable to Step:
|
|
// *AuthStep_Choice_
|
|
// *AuthStep_Form_
|
|
// *AuthStep_Session
|
|
// *AuthStep_Waiting_
|
|
Step isAuthStep_Step `protobuf_oneof:"step"`
|
|
}
|
|
|
|
func (x *AuthStep) Reset() {
|
|
*x = AuthStep{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *AuthStep) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AuthStep) ProtoMessage() {}
|
|
|
|
func (x *AuthStep) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[3]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AuthStep.ProtoReflect.Descriptor instead.
|
|
func (*AuthStep) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *AuthStep) GetFallbackUrl() string {
|
|
if x != nil {
|
|
return x.FallbackUrl
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AuthStep) GetCanGoBack() bool {
|
|
if x != nil {
|
|
return x.CanGoBack
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (m *AuthStep) GetStep() isAuthStep_Step {
|
|
if m != nil {
|
|
return m.Step
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *AuthStep) GetChoice() *AuthStep_Choice {
|
|
if x, ok := x.GetStep().(*AuthStep_Choice_); ok {
|
|
return x.Choice
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *AuthStep) GetForm() *AuthStep_Form {
|
|
if x, ok := x.GetStep().(*AuthStep_Form_); ok {
|
|
return x.Form
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *AuthStep) GetSession() *Session {
|
|
if x, ok := x.GetStep().(*AuthStep_Session); ok {
|
|
return x.Session
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *AuthStep) GetWaiting() *AuthStep_Waiting {
|
|
if x, ok := x.GetStep().(*AuthStep_Waiting_); ok {
|
|
return x.Waiting
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type isAuthStep_Step interface {
|
|
isAuthStep_Step()
|
|
}
|
|
|
|
type AuthStep_Choice_ struct {
|
|
// choice: the user must pick a thing out of a list of options
|
|
Choice *AuthStep_Choice `protobuf:"bytes,3,opt,name=choice,proto3,oneof"`
|
|
}
|
|
|
|
type AuthStep_Form_ struct {
|
|
// form: the user must complete a form
|
|
Form *AuthStep_Form `protobuf:"bytes,4,opt,name=form,proto3,oneof"`
|
|
}
|
|
|
|
type AuthStep_Session struct {
|
|
// session: you've completed auth, and have a session
|
|
Session *Session `protobuf:"bytes,5,opt,name=session,proto3,oneof"`
|
|
}
|
|
|
|
type AuthStep_Waiting_ struct {
|
|
// waiting: you're waiting on something
|
|
Waiting *AuthStep_Waiting `protobuf:"bytes,6,opt,name=waiting,proto3,oneof"`
|
|
}
|
|
|
|
func (*AuthStep_Choice_) isAuthStep_Step() {}
|
|
|
|
func (*AuthStep_Form_) isAuthStep_Step() {}
|
|
|
|
func (*AuthStep_Session) isAuthStep_Step() {}
|
|
|
|
func (*AuthStep_Waiting_) isAuthStep_Step() {}
|
|
|
|
// NextStepRequest
|
|
// contains the client's response to the server's challenge
|
|
// This needs to be called first with no arguments to
|
|
// receive the first step
|
|
type NextStepRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// auth_id: the authentication session you want
|
|
// the next step of
|
|
AuthId string `protobuf:"bytes,1,opt,name=auth_id,json=authId,proto3" json:"auth_id,omitempty"`
|
|
// step: the user's response to a step
|
|
//
|
|
// Types that are assignable to Step:
|
|
// *NextStepRequest_Choice_
|
|
// *NextStepRequest_Form_
|
|
Step isNextStepRequest_Step `protobuf_oneof:"step"`
|
|
}
|
|
|
|
func (x *NextStepRequest) Reset() {
|
|
*x = NextStepRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NextStepRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NextStepRequest) ProtoMessage() {}
|
|
|
|
func (x *NextStepRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[4]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NextStepRequest.ProtoReflect.Descriptor instead.
|
|
func (*NextStepRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
func (x *NextStepRequest) GetAuthId() string {
|
|
if x != nil {
|
|
return x.AuthId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *NextStepRequest) GetStep() isNextStepRequest_Step {
|
|
if m != nil {
|
|
return m.Step
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *NextStepRequest) GetChoice() *NextStepRequest_Choice {
|
|
if x, ok := x.GetStep().(*NextStepRequest_Choice_); ok {
|
|
return x.Choice
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *NextStepRequest) GetForm() *NextStepRequest_Form {
|
|
if x, ok := x.GetStep().(*NextStepRequest_Form_); ok {
|
|
return x.Form
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type isNextStepRequest_Step interface {
|
|
isNextStepRequest_Step()
|
|
}
|
|
|
|
type NextStepRequest_Choice_ struct {
|
|
// choice: the choice the user picked
|
|
Choice *NextStepRequest_Choice `protobuf:"bytes,2,opt,name=choice,proto3,oneof"`
|
|
}
|
|
|
|
type NextStepRequest_Form_ struct {
|
|
// form: the form the user filled out
|
|
Form *NextStepRequest_Form `protobuf:"bytes,3,opt,name=form,proto3,oneof"`
|
|
}
|
|
|
|
func (*NextStepRequest_Choice_) isNextStepRequest_Step() {}
|
|
|
|
func (*NextStepRequest_Form_) isNextStepRequest_Step() {}
|
|
|
|
// Used in `NextStep` endpoint.
|
|
type NextStepResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// step: the next step in the authentication process
|
|
Step *AuthStep `protobuf:"bytes,1,opt,name=step,proto3" json:"step,omitempty"`
|
|
}
|
|
|
|
func (x *NextStepResponse) Reset() {
|
|
*x = NextStepResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NextStepResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NextStepResponse) ProtoMessage() {}
|
|
|
|
func (x *NextStepResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[5]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NextStepResponse.ProtoReflect.Descriptor instead.
|
|
func (*NextStepResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *NextStepResponse) GetStep() *AuthStep {
|
|
if x != nil {
|
|
return x.Step
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// StepBackRequest
|
|
// A request to go back 1 step
|
|
type StepBackRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// auth_id: the authentication session the user
|
|
// wants to go back in
|
|
AuthId string `protobuf:"bytes,1,opt,name=auth_id,json=authId,proto3" json:"auth_id,omitempty"`
|
|
}
|
|
|
|
func (x *StepBackRequest) Reset() {
|
|
*x = StepBackRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StepBackRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StepBackRequest) ProtoMessage() {}
|
|
|
|
func (x *StepBackRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[6]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StepBackRequest.ProtoReflect.Descriptor instead.
|
|
func (*StepBackRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *StepBackRequest) GetAuthId() string {
|
|
if x != nil {
|
|
return x.AuthId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Used in `StepBack` endpoint.
|
|
type StepBackResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// step: the previous step in the authentication process
|
|
Step *AuthStep `protobuf:"bytes,1,opt,name=step,proto3" json:"step,omitempty"`
|
|
}
|
|
|
|
func (x *StepBackResponse) Reset() {
|
|
*x = StepBackResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StepBackResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StepBackResponse) ProtoMessage() {}
|
|
|
|
func (x *StepBackResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[7]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StepBackResponse.ProtoReflect.Descriptor instead.
|
|
func (*StepBackResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *StepBackResponse) GetStep() *AuthStep {
|
|
if x != nil {
|
|
return x.Step
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// StreamStepsRequest
|
|
// Required to be initiated by all authenticating clients
|
|
// Allows the server to send steps
|
|
type StreamStepsRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// auth_id: the authorization session
|
|
// who's steps you want to stream
|
|
AuthId string `protobuf:"bytes,1,opt,name=auth_id,json=authId,proto3" json:"auth_id,omitempty"`
|
|
}
|
|
|
|
func (x *StreamStepsRequest) Reset() {
|
|
*x = StreamStepsRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StreamStepsRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StreamStepsRequest) ProtoMessage() {}
|
|
|
|
func (x *StreamStepsRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[8]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StreamStepsRequest.ProtoReflect.Descriptor instead.
|
|
func (*StreamStepsRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *StreamStepsRequest) GetAuthId() string {
|
|
if x != nil {
|
|
return x.AuthId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Used in `StreamSteps` endpoint.
|
|
type StreamStepsResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// step: the next step in the authentication process
|
|
Step *AuthStep `protobuf:"bytes,1,opt,name=step,proto3" json:"step,omitempty"`
|
|
}
|
|
|
|
func (x *StreamStepsResponse) Reset() {
|
|
*x = StreamStepsResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[9]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StreamStepsResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StreamStepsResponse) ProtoMessage() {}
|
|
|
|
func (x *StreamStepsResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[9]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StreamStepsResponse.ProtoReflect.Descriptor instead.
|
|
func (*StreamStepsResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{9}
|
|
}
|
|
|
|
func (x *StreamStepsResponse) GetStep() *AuthStep {
|
|
if x != nil {
|
|
return x.Step
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// The request to federate with a foreign server.
|
|
type FederateRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// The server ID foreign server you want to federate with
|
|
ServerId string `protobuf:"bytes,1,opt,name=server_id,json=serverId,proto3" json:"server_id,omitempty"`
|
|
}
|
|
|
|
func (x *FederateRequest) Reset() {
|
|
*x = FederateRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[10]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *FederateRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*FederateRequest) ProtoMessage() {}
|
|
|
|
func (x *FederateRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[10]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use FederateRequest.ProtoReflect.Descriptor instead.
|
|
func (*FederateRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{10}
|
|
}
|
|
|
|
func (x *FederateRequest) GetServerId() string {
|
|
if x != nil {
|
|
return x.ServerId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// The reply to a successful federation request,
|
|
// containing the token you need to present to the
|
|
// foreign server.
|
|
type FederateResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// A `harmonytypes.v1.Token` whose `data` field is a serialized `TokenData` message.
|
|
// It is signed with the homeserver's private key.
|
|
Token *v1.Token `protobuf:"bytes,1,opt,name=token,proto3" json:"token,omitempty"`
|
|
}
|
|
|
|
func (x *FederateResponse) Reset() {
|
|
*x = FederateResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[11]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *FederateResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*FederateResponse) ProtoMessage() {}
|
|
|
|
func (x *FederateResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[11]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use FederateResponse.ProtoReflect.Descriptor instead.
|
|
func (*FederateResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{11}
|
|
}
|
|
|
|
func (x *FederateResponse) GetToken() *v1.Token {
|
|
if x != nil {
|
|
return x.Token
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Used in `Key` endpoint.
|
|
type KeyRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *KeyRequest) Reset() {
|
|
*x = KeyRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[12]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *KeyRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*KeyRequest) ProtoMessage() {}
|
|
|
|
func (x *KeyRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[12]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use KeyRequest.ProtoReflect.Descriptor instead.
|
|
func (*KeyRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{12}
|
|
}
|
|
|
|
// Contains a key's bytes.
|
|
type KeyResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// key: the bytes of the public key.
|
|
Key []byte `protobuf:"bytes,1,opt,name=key,proto3" json:"key,omitempty"`
|
|
}
|
|
|
|
func (x *KeyResponse) Reset() {
|
|
*x = KeyResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[13]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *KeyResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*KeyResponse) ProtoMessage() {}
|
|
|
|
func (x *KeyResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[13]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use KeyResponse.ProtoReflect.Descriptor instead.
|
|
func (*KeyResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{13}
|
|
}
|
|
|
|
func (x *KeyResponse) GetKey() []byte {
|
|
if x != nil {
|
|
return x.Key
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Log into a foreignserver using a token
|
|
// from your homeserver, obtained through a FederateRequest
|
|
type LoginFederatedRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// A `harmonytypes.v1.Token` whose `data` field is a serialized `TokenData` message.
|
|
// It is signed with the homeserver's private key.
|
|
AuthToken *v1.Token `protobuf:"bytes,1,opt,name=auth_token,json=authToken,proto3" json:"auth_token,omitempty"`
|
|
// The server ID of the homeserver that the auth token is from
|
|
ServerId string `protobuf:"bytes,2,opt,name=server_id,json=serverId,proto3" json:"server_id,omitempty"`
|
|
}
|
|
|
|
func (x *LoginFederatedRequest) Reset() {
|
|
*x = LoginFederatedRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[14]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *LoginFederatedRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*LoginFederatedRequest) ProtoMessage() {}
|
|
|
|
func (x *LoginFederatedRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[14]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use LoginFederatedRequest.ProtoReflect.Descriptor instead.
|
|
func (*LoginFederatedRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{14}
|
|
}
|
|
|
|
func (x *LoginFederatedRequest) GetAuthToken() *v1.Token {
|
|
if x != nil {
|
|
return x.AuthToken
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *LoginFederatedRequest) GetServerId() string {
|
|
if x != nil {
|
|
return x.ServerId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Used in `LoginFederated` endpoint.
|
|
type LoginFederatedResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// The user's session.
|
|
Session *Session `protobuf:"bytes,1,opt,name=session,proto3" json:"session,omitempty"`
|
|
}
|
|
|
|
func (x *LoginFederatedResponse) Reset() {
|
|
*x = LoginFederatedResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[15]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *LoginFederatedResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*LoginFederatedResponse) ProtoMessage() {}
|
|
|
|
func (x *LoginFederatedResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[15]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use LoginFederatedResponse.ProtoReflect.Descriptor instead.
|
|
func (*LoginFederatedResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{15}
|
|
}
|
|
|
|
func (x *LoginFederatedResponse) GetSession() *Session {
|
|
if x != nil {
|
|
return x.Session
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Information sent by a client's homeserver, in a `harmonytypes.v1.Token`.
|
|
// It will be sent to a foreignserver by the client.
|
|
type TokenData struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// The client's user ID on the homeserver.
|
|
UserId uint64 `protobuf:"varint,1,opt,name=user_id,json=userId,proto3" json:"user_id,omitempty"`
|
|
// The foreignserver's server ID.
|
|
ServerId string `protobuf:"bytes,2,opt,name=server_id,json=serverId,proto3" json:"server_id,omitempty"`
|
|
// The username of the client.
|
|
Username string `protobuf:"bytes,3,opt,name=username,proto3" json:"username,omitempty"`
|
|
// The avatar of the client.
|
|
Avatar *string `protobuf:"bytes,4,opt,name=avatar,proto3,oneof" json:"avatar,omitempty"`
|
|
}
|
|
|
|
func (x *TokenData) Reset() {
|
|
*x = TokenData{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[16]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *TokenData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TokenData) ProtoMessage() {}
|
|
|
|
func (x *TokenData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[16]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TokenData.ProtoReflect.Descriptor instead.
|
|
func (*TokenData) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{16}
|
|
}
|
|
|
|
func (x *TokenData) GetUserId() uint64 {
|
|
if x != nil {
|
|
return x.UserId
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *TokenData) GetServerId() string {
|
|
if x != nil {
|
|
return x.ServerId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *TokenData) GetUsername() string {
|
|
if x != nil {
|
|
return x.Username
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *TokenData) GetAvatar() string {
|
|
if x != nil && x.Avatar != nil {
|
|
return *x.Avatar
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Used in `CheckLoggedIn` endpoint.
|
|
type CheckLoggedInRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *CheckLoggedInRequest) Reset() {
|
|
*x = CheckLoggedInRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[17]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *CheckLoggedInRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*CheckLoggedInRequest) ProtoMessage() {}
|
|
|
|
func (x *CheckLoggedInRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[17]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use CheckLoggedInRequest.ProtoReflect.Descriptor instead.
|
|
func (*CheckLoggedInRequest) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{17}
|
|
}
|
|
|
|
// Used in `CheckLoggedIn` endpoint.
|
|
type CheckLoggedInResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *CheckLoggedInResponse) Reset() {
|
|
*x = CheckLoggedInResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[18]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *CheckLoggedInResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*CheckLoggedInResponse) ProtoMessage() {}
|
|
|
|
func (x *CheckLoggedInResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[18]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use CheckLoggedInResponse.ProtoReflect.Descriptor instead.
|
|
func (*CheckLoggedInResponse) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{18}
|
|
}
|
|
|
|
// Choice
|
|
// A step which allows the user to choose from a range of options
|
|
// Allows you to show a heading by specifying title
|
|
type AuthStep_Choice struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// title: the title of the list of choices
|
|
Title string `protobuf:"bytes,1,opt,name=title,proto3" json:"title,omitempty"`
|
|
// options: a list of choices, one of these
|
|
// should be sent in nextstep
|
|
Options []string `protobuf:"bytes,2,rep,name=options,proto3" json:"options,omitempty"`
|
|
}
|
|
|
|
func (x *AuthStep_Choice) Reset() {
|
|
*x = AuthStep_Choice{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[19]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *AuthStep_Choice) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AuthStep_Choice) ProtoMessage() {}
|
|
|
|
func (x *AuthStep_Choice) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[19]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AuthStep_Choice.ProtoReflect.Descriptor instead.
|
|
func (*AuthStep_Choice) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{3, 0}
|
|
}
|
|
|
|
func (x *AuthStep_Choice) GetTitle() string {
|
|
if x != nil {
|
|
return x.Title
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AuthStep_Choice) GetOptions() []string {
|
|
if x != nil {
|
|
return x.Options
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Form
|
|
// A step which requires the user to input information
|
|
// Allows you to show a heading by specifying title
|
|
type AuthStep_Form struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// title: the title of this form
|
|
Title string `protobuf:"bytes,1,opt,name=title,proto3" json:"title,omitempty"`
|
|
// fields: all the fields in this form
|
|
Fields []*AuthStep_Form_FormField `protobuf:"bytes,2,rep,name=fields,proto3" json:"fields,omitempty"`
|
|
}
|
|
|
|
func (x *AuthStep_Form) Reset() {
|
|
*x = AuthStep_Form{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[20]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *AuthStep_Form) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AuthStep_Form) ProtoMessage() {}
|
|
|
|
func (x *AuthStep_Form) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[20]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AuthStep_Form.ProtoReflect.Descriptor instead.
|
|
func (*AuthStep_Form) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{3, 1}
|
|
}
|
|
|
|
func (x *AuthStep_Form) GetTitle() string {
|
|
if x != nil {
|
|
return x.Title
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AuthStep_Form) GetFields() []*AuthStep_Form_FormField {
|
|
if x != nil {
|
|
return x.Fields
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Waiting
|
|
// A step which requires the user to perform an external action
|
|
// The title and description should explain to the user
|
|
// what they should do to complete this step
|
|
type AuthStep_Waiting struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// title: the title of this waiting screen
|
|
Title string `protobuf:"bytes,1,opt,name=title,proto3" json:"title,omitempty"`
|
|
// description: the explanation of what's being waited on
|
|
Description string `protobuf:"bytes,2,opt,name=description,proto3" json:"description,omitempty"`
|
|
}
|
|
|
|
func (x *AuthStep_Waiting) Reset() {
|
|
*x = AuthStep_Waiting{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[21]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *AuthStep_Waiting) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AuthStep_Waiting) ProtoMessage() {}
|
|
|
|
func (x *AuthStep_Waiting) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[21]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AuthStep_Waiting.ProtoReflect.Descriptor instead.
|
|
func (*AuthStep_Waiting) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{3, 2}
|
|
}
|
|
|
|
func (x *AuthStep_Waiting) GetTitle() string {
|
|
if x != nil {
|
|
return x.Title
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AuthStep_Waiting) GetDescription() string {
|
|
if x != nil {
|
|
return x.Description
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// FormField
|
|
// A field in the form, containing information on how it should
|
|
// be rendered
|
|
// Here is a list of form types that need to be supported:
|
|
// email: a field type that has to contain a valid email
|
|
// password: a field type that has to contain a password
|
|
// new-password: a field type for new passwords
|
|
// text: a field type that has to contain text
|
|
// number: a field type that has to contain a number
|
|
type AuthStep_Form_FormField struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// name: the identifier for the form field
|
|
Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"`
|
|
// type: the type of the form field, as documented above
|
|
Type string `protobuf:"bytes,2,opt,name=type,proto3" json:"type,omitempty"`
|
|
}
|
|
|
|
func (x *AuthStep_Form_FormField) Reset() {
|
|
*x = AuthStep_Form_FormField{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[22]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *AuthStep_Form_FormField) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AuthStep_Form_FormField) ProtoMessage() {}
|
|
|
|
func (x *AuthStep_Form_FormField) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[22]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AuthStep_Form_FormField.ProtoReflect.Descriptor instead.
|
|
func (*AuthStep_Form_FormField) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{3, 1, 0}
|
|
}
|
|
|
|
func (x *AuthStep_Form_FormField) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AuthStep_Form_FormField) GetType() string {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// A simple choice string indicating which option the user chose
|
|
type NextStepRequest_Choice struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// choice: the choice the user picked
|
|
Choice string `protobuf:"bytes,1,opt,name=choice,proto3" json:"choice,omitempty"`
|
|
}
|
|
|
|
func (x *NextStepRequest_Choice) Reset() {
|
|
*x = NextStepRequest_Choice{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[23]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NextStepRequest_Choice) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NextStepRequest_Choice) ProtoMessage() {}
|
|
|
|
func (x *NextStepRequest_Choice) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[23]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NextStepRequest_Choice.ProtoReflect.Descriptor instead.
|
|
func (*NextStepRequest_Choice) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{4, 0}
|
|
}
|
|
|
|
func (x *NextStepRequest_Choice) GetChoice() string {
|
|
if x != nil {
|
|
return x.Choice
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Form fields can either be bytes, string, or int64.
|
|
type NextStepRequest_FormFields struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// field: the data for a form field
|
|
//
|
|
// Types that are assignable to Field:
|
|
// *NextStepRequest_FormFields_Bytes
|
|
// *NextStepRequest_FormFields_String_
|
|
// *NextStepRequest_FormFields_Number
|
|
Field isNextStepRequest_FormFields_Field `protobuf_oneof:"field"`
|
|
}
|
|
|
|
func (x *NextStepRequest_FormFields) Reset() {
|
|
*x = NextStepRequest_FormFields{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[24]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NextStepRequest_FormFields) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NextStepRequest_FormFields) ProtoMessage() {}
|
|
|
|
func (x *NextStepRequest_FormFields) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[24]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NextStepRequest_FormFields.ProtoReflect.Descriptor instead.
|
|
func (*NextStepRequest_FormFields) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{4, 1}
|
|
}
|
|
|
|
func (m *NextStepRequest_FormFields) GetField() isNextStepRequest_FormFields_Field {
|
|
if m != nil {
|
|
return m.Field
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *NextStepRequest_FormFields) GetBytes() []byte {
|
|
if x, ok := x.GetField().(*NextStepRequest_FormFields_Bytes); ok {
|
|
return x.Bytes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *NextStepRequest_FormFields) GetString_() string {
|
|
if x, ok := x.GetField().(*NextStepRequest_FormFields_String_); ok {
|
|
return x.String_
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *NextStepRequest_FormFields) GetNumber() int64 {
|
|
if x, ok := x.GetField().(*NextStepRequest_FormFields_Number); ok {
|
|
return x.Number
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type isNextStepRequest_FormFields_Field interface {
|
|
isNextStepRequest_FormFields_Field()
|
|
}
|
|
|
|
type NextStepRequest_FormFields_Bytes struct {
|
|
// bytes: the form field's data is a byte array
|
|
Bytes []byte `protobuf:"bytes,1,opt,name=bytes,proto3,oneof"`
|
|
}
|
|
|
|
type NextStepRequest_FormFields_String_ struct {
|
|
// string: the form field's data is a string
|
|
String_ string `protobuf:"bytes,2,opt,name=string,proto3,oneof"`
|
|
}
|
|
|
|
type NextStepRequest_FormFields_Number struct {
|
|
// number: the form field's data is a number
|
|
Number int64 `protobuf:"varint,3,opt,name=number,proto3,oneof"`
|
|
}
|
|
|
|
func (*NextStepRequest_FormFields_Bytes) isNextStepRequest_FormFields_Field() {}
|
|
|
|
func (*NextStepRequest_FormFields_String_) isNextStepRequest_FormFields_Field() {}
|
|
|
|
func (*NextStepRequest_FormFields_Number) isNextStepRequest_FormFields_Field() {}
|
|
|
|
// An array of form fields, in the same order they came in from the server
|
|
type NextStepRequest_Form struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// fields: the fields the user filled out
|
|
Fields []*NextStepRequest_FormFields `protobuf:"bytes,1,rep,name=fields,proto3" json:"fields,omitempty"`
|
|
}
|
|
|
|
func (x *NextStepRequest_Form) Reset() {
|
|
*x = NextStepRequest_Form{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[25]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NextStepRequest_Form) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NextStepRequest_Form) ProtoMessage() {}
|
|
|
|
func (x *NextStepRequest_Form) ProtoReflect() protoreflect.Message {
|
|
mi := &file_auth_v1_auth_proto_msgTypes[25]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NextStepRequest_Form.ProtoReflect.Descriptor instead.
|
|
func (*NextStepRequest_Form) Descriptor() ([]byte, []int) {
|
|
return file_auth_v1_auth_proto_rawDescGZIP(), []int{4, 2}
|
|
}
|
|
|
|
func (x *NextStepRequest_Form) GetFields() []*NextStepRequest_FormFields {
|
|
if x != nil {
|
|
return x.Fields
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_auth_v1_auth_proto protoreflect.FileDescriptor
|
|
|
|
var file_auth_v1_auth_proto_rawDesc = []byte{
|
|
0x0a, 0x12, 0x61, 0x75, 0x74, 0x68, 0x2f, 0x76, 0x31, 0x2f, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x12, 0x10, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61,
|
|
0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x1a, 0x1b, 0x68, 0x61, 0x72, 0x6d, 0x6f, 0x6e, 0x79, 0x74,
|
|
0x79, 0x70, 0x65, 0x73, 0x2f, 0x76, 0x31, 0x2f, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x22, 0x12, 0x0a, 0x10, 0x42, 0x65, 0x67, 0x69, 0x6e, 0x41, 0x75, 0x74, 0x68,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x2c, 0x0a, 0x11, 0x42, 0x65, 0x67, 0x69, 0x6e,
|
|
0x41, 0x75, 0x74, 0x68, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x17, 0x0a, 0x07,
|
|
0x61, 0x75, 0x74, 0x68, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x61,
|
|
0x75, 0x74, 0x68, 0x49, 0x64, 0x22, 0x47, 0x0a, 0x07, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e,
|
|
0x12, 0x17, 0x0a, 0x07, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x06, 0x75, 0x73, 0x65, 0x72, 0x49, 0x64, 0x12, 0x23, 0x0a, 0x0d, 0x73, 0x65, 0x73,
|
|
0x73, 0x69, 0x6f, 0x6e, 0x5f, 0x74, 0x6f, 0x6b, 0x65, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x0c, 0x73, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x54, 0x6f, 0x6b, 0x65, 0x6e, 0x22, 0xd4,
|
|
0x04, 0x0a, 0x08, 0x41, 0x75, 0x74, 0x68, 0x53, 0x74, 0x65, 0x70, 0x12, 0x21, 0x0a, 0x0c, 0x66,
|
|
0x61, 0x6c, 0x6c, 0x62, 0x61, 0x63, 0x6b, 0x5f, 0x75, 0x72, 0x6c, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x0b, 0x66, 0x61, 0x6c, 0x6c, 0x62, 0x61, 0x63, 0x6b, 0x55, 0x72, 0x6c, 0x12, 0x1e,
|
|
0x0a, 0x0b, 0x63, 0x61, 0x6e, 0x5f, 0x67, 0x6f, 0x5f, 0x62, 0x61, 0x63, 0x6b, 0x18, 0x02, 0x20,
|
|
0x01, 0x28, 0x08, 0x52, 0x09, 0x63, 0x61, 0x6e, 0x47, 0x6f, 0x42, 0x61, 0x63, 0x6b, 0x12, 0x3b,
|
|
0x0a, 0x06, 0x63, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x21,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x41, 0x75, 0x74, 0x68, 0x53, 0x74, 0x65, 0x70, 0x2e, 0x43, 0x68, 0x6f, 0x69, 0x63,
|
|
0x65, 0x48, 0x00, 0x52, 0x06, 0x63, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x12, 0x35, 0x0a, 0x04, 0x66,
|
|
0x6f, 0x72, 0x6d, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1f, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x75, 0x74,
|
|
0x68, 0x53, 0x74, 0x65, 0x70, 0x2e, 0x46, 0x6f, 0x72, 0x6d, 0x48, 0x00, 0x52, 0x04, 0x66, 0x6f,
|
|
0x72, 0x6d, 0x12, 0x35, 0x0a, 0x07, 0x73, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61,
|
|
0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x48, 0x00,
|
|
0x52, 0x07, 0x73, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x3e, 0x0a, 0x07, 0x77, 0x61, 0x69,
|
|
0x74, 0x69, 0x6e, 0x67, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x22, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x75,
|
|
0x74, 0x68, 0x53, 0x74, 0x65, 0x70, 0x2e, 0x57, 0x61, 0x69, 0x74, 0x69, 0x6e, 0x67, 0x48, 0x00,
|
|
0x52, 0x07, 0x77, 0x61, 0x69, 0x74, 0x69, 0x6e, 0x67, 0x1a, 0x38, 0x0a, 0x06, 0x43, 0x68, 0x6f,
|
|
0x69, 0x63, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x12, 0x18, 0x0a, 0x07, 0x6f, 0x70, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x07, 0x6f, 0x70, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x73, 0x1a, 0x94, 0x01, 0x0a, 0x04, 0x46, 0x6f, 0x72, 0x6d, 0x12, 0x14, 0x0a, 0x05,
|
|
0x74, 0x69, 0x74, 0x6c, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x74, 0x69, 0x74,
|
|
0x6c, 0x65, 0x12, 0x41, 0x0a, 0x06, 0x66, 0x69, 0x65, 0x6c, 0x64, 0x73, 0x18, 0x02, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x29, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75,
|
|
0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x75, 0x74, 0x68, 0x53, 0x74, 0x65, 0x70, 0x2e, 0x46,
|
|
0x6f, 0x72, 0x6d, 0x2e, 0x46, 0x6f, 0x72, 0x6d, 0x46, 0x69, 0x65, 0x6c, 0x64, 0x52, 0x06, 0x66,
|
|
0x69, 0x65, 0x6c, 0x64, 0x73, 0x1a, 0x33, 0x0a, 0x09, 0x46, 0x6f, 0x72, 0x6d, 0x46, 0x69, 0x65,
|
|
0x6c, 0x64, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x1a, 0x41, 0x0a, 0x07, 0x57, 0x61,
|
|
0x69, 0x74, 0x69, 0x6e, 0x67, 0x12, 0x14, 0x0a, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x74, 0x69, 0x74, 0x6c, 0x65, 0x12, 0x20, 0x0a, 0x0b, 0x64,
|
|
0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x0b, 0x64, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x42, 0x06, 0x0a,
|
|
0x04, 0x73, 0x74, 0x65, 0x70, 0x22, 0x87, 0x03, 0x0a, 0x0f, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74,
|
|
0x65, 0x70, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x17, 0x0a, 0x07, 0x61, 0x75, 0x74,
|
|
0x68, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x61, 0x75, 0x74, 0x68,
|
|
0x49, 0x64, 0x12, 0x42, 0x0a, 0x06, 0x63, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x28, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75,
|
|
0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74, 0x65, 0x70, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x2e, 0x43, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x48, 0x00, 0x52, 0x06,
|
|
0x63, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x12, 0x3c, 0x0a, 0x04, 0x66, 0x6f, 0x72, 0x6d, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x26, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e,
|
|
0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74, 0x65, 0x70,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x2e, 0x46, 0x6f, 0x72, 0x6d, 0x48, 0x00, 0x52, 0x04,
|
|
0x66, 0x6f, 0x72, 0x6d, 0x1a, 0x20, 0x0a, 0x06, 0x43, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x12, 0x16,
|
|
0x0a, 0x06, 0x63, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06,
|
|
0x63, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x1a, 0x61, 0x0a, 0x0a, 0x46, 0x6f, 0x72, 0x6d, 0x46, 0x69,
|
|
0x65, 0x6c, 0x64, 0x73, 0x12, 0x16, 0x0a, 0x05, 0x62, 0x79, 0x74, 0x65, 0x73, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x0c, 0x48, 0x00, 0x52, 0x05, 0x62, 0x79, 0x74, 0x65, 0x73, 0x12, 0x18, 0x0a, 0x06,
|
|
0x73, 0x74, 0x72, 0x69, 0x6e, 0x67, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x48, 0x00, 0x52, 0x06,
|
|
0x73, 0x74, 0x72, 0x69, 0x6e, 0x67, 0x12, 0x18, 0x0a, 0x06, 0x6e, 0x75, 0x6d, 0x62, 0x65, 0x72,
|
|
0x18, 0x03, 0x20, 0x01, 0x28, 0x03, 0x48, 0x00, 0x52, 0x06, 0x6e, 0x75, 0x6d, 0x62, 0x65, 0x72,
|
|
0x42, 0x07, 0x0a, 0x05, 0x66, 0x69, 0x65, 0x6c, 0x64, 0x1a, 0x4c, 0x0a, 0x04, 0x46, 0x6f, 0x72,
|
|
0x6d, 0x12, 0x44, 0x0a, 0x06, 0x66, 0x69, 0x65, 0x6c, 0x64, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x2c, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74,
|
|
0x68, 0x2e, 0x76, 0x31, 0x2e, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74, 0x65, 0x70, 0x52, 0x65, 0x71,
|
|
0x75, 0x65, 0x73, 0x74, 0x2e, 0x46, 0x6f, 0x72, 0x6d, 0x46, 0x69, 0x65, 0x6c, 0x64, 0x73, 0x52,
|
|
0x06, 0x66, 0x69, 0x65, 0x6c, 0x64, 0x73, 0x42, 0x06, 0x0a, 0x04, 0x73, 0x74, 0x65, 0x70, 0x22,
|
|
0x42, 0x0a, 0x10, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74, 0x65, 0x70, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x12, 0x2e, 0x0a, 0x04, 0x73, 0x74, 0x65, 0x70, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1a, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74,
|
|
0x68, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x75, 0x74, 0x68, 0x53, 0x74, 0x65, 0x70, 0x52, 0x04, 0x73,
|
|
0x74, 0x65, 0x70, 0x22, 0x2a, 0x0a, 0x0f, 0x53, 0x74, 0x65, 0x70, 0x42, 0x61, 0x63, 0x6b, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x17, 0x0a, 0x07, 0x61, 0x75, 0x74, 0x68, 0x5f, 0x69,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x61, 0x75, 0x74, 0x68, 0x49, 0x64, 0x22,
|
|
0x42, 0x0a, 0x10, 0x53, 0x74, 0x65, 0x70, 0x42, 0x61, 0x63, 0x6b, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x12, 0x2e, 0x0a, 0x04, 0x73, 0x74, 0x65, 0x70, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1a, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74,
|
|
0x68, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x75, 0x74, 0x68, 0x53, 0x74, 0x65, 0x70, 0x52, 0x04, 0x73,
|
|
0x74, 0x65, 0x70, 0x22, 0x2d, 0x0a, 0x12, 0x53, 0x74, 0x72, 0x65, 0x61, 0x6d, 0x53, 0x74, 0x65,
|
|
0x70, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x17, 0x0a, 0x07, 0x61, 0x75, 0x74,
|
|
0x68, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x61, 0x75, 0x74, 0x68,
|
|
0x49, 0x64, 0x22, 0x45, 0x0a, 0x13, 0x53, 0x74, 0x72, 0x65, 0x61, 0x6d, 0x53, 0x74, 0x65, 0x70,
|
|
0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x2e, 0x0a, 0x04, 0x73, 0x74, 0x65,
|
|
0x70, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63,
|
|
0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x41, 0x75, 0x74, 0x68, 0x53,
|
|
0x74, 0x65, 0x70, 0x52, 0x04, 0x73, 0x74, 0x65, 0x70, 0x22, 0x2e, 0x0a, 0x0f, 0x46, 0x65, 0x64,
|
|
0x65, 0x72, 0x61, 0x74, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x1b, 0x0a, 0x09,
|
|
0x73, 0x65, 0x72, 0x76, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x08, 0x73, 0x65, 0x72, 0x76, 0x65, 0x72, 0x49, 0x64, 0x22, 0x49, 0x0a, 0x10, 0x46, 0x65, 0x64,
|
|
0x65, 0x72, 0x61, 0x74, 0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x35, 0x0a,
|
|
0x05, 0x74, 0x6f, 0x6b, 0x65, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1f, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x68, 0x61, 0x72, 0x6d, 0x6f, 0x6e, 0x79, 0x74,
|
|
0x79, 0x70, 0x65, 0x73, 0x2e, 0x76, 0x31, 0x2e, 0x54, 0x6f, 0x6b, 0x65, 0x6e, 0x52, 0x05, 0x74,
|
|
0x6f, 0x6b, 0x65, 0x6e, 0x22, 0x0c, 0x0a, 0x0a, 0x4b, 0x65, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x22, 0x1f, 0x0a, 0x0b, 0x4b, 0x65, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73,
|
|
0x65, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x03,
|
|
0x6b, 0x65, 0x79, 0x22, 0x74, 0x0a, 0x15, 0x4c, 0x6f, 0x67, 0x69, 0x6e, 0x46, 0x65, 0x64, 0x65,
|
|
0x72, 0x61, 0x74, 0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x3e, 0x0a, 0x0a,
|
|
0x61, 0x75, 0x74, 0x68, 0x5f, 0x74, 0x6f, 0x6b, 0x65, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x1f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x68, 0x61, 0x72, 0x6d,
|
|
0x6f, 0x6e, 0x79, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x76, 0x31, 0x2e, 0x54, 0x6f, 0x6b, 0x65,
|
|
0x6e, 0x52, 0x09, 0x61, 0x75, 0x74, 0x68, 0x54, 0x6f, 0x6b, 0x65, 0x6e, 0x12, 0x1b, 0x0a, 0x09,
|
|
0x73, 0x65, 0x72, 0x76, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x08, 0x73, 0x65, 0x72, 0x76, 0x65, 0x72, 0x49, 0x64, 0x22, 0x4d, 0x0a, 0x16, 0x4c, 0x6f, 0x67,
|
|
0x69, 0x6e, 0x46, 0x65, 0x64, 0x65, 0x72, 0x61, 0x74, 0x65, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x12, 0x33, 0x0a, 0x07, 0x73, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e,
|
|
0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x52,
|
|
0x07, 0x73, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x22, 0x85, 0x01, 0x0a, 0x09, 0x54, 0x6f, 0x6b,
|
|
0x65, 0x6e, 0x44, 0x61, 0x74, 0x61, 0x12, 0x17, 0x0a, 0x07, 0x75, 0x73, 0x65, 0x72, 0x5f, 0x69,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x06, 0x75, 0x73, 0x65, 0x72, 0x49, 0x64, 0x12,
|
|
0x1b, 0x0a, 0x09, 0x73, 0x65, 0x72, 0x76, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x08, 0x73, 0x65, 0x72, 0x76, 0x65, 0x72, 0x49, 0x64, 0x12, 0x1a, 0x0a, 0x08,
|
|
0x75, 0x73, 0x65, 0x72, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08,
|
|
0x75, 0x73, 0x65, 0x72, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x1b, 0x0a, 0x06, 0x61, 0x76, 0x61, 0x74,
|
|
0x61, 0x72, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x48, 0x00, 0x52, 0x06, 0x61, 0x76, 0x61, 0x74,
|
|
0x61, 0x72, 0x88, 0x01, 0x01, 0x42, 0x09, 0x0a, 0x07, 0x5f, 0x61, 0x76, 0x61, 0x74, 0x61, 0x72,
|
|
0x22, 0x16, 0x0a, 0x14, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x4c, 0x6f, 0x67, 0x67, 0x65, 0x64, 0x49,
|
|
0x6e, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x17, 0x0a, 0x15, 0x43, 0x68, 0x65, 0x63,
|
|
0x6b, 0x4c, 0x6f, 0x67, 0x67, 0x65, 0x64, 0x49, 0x6e, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73,
|
|
0x65, 0x32, 0xcc, 0x05, 0x0a, 0x0b, 0x41, 0x75, 0x74, 0x68, 0x53, 0x65, 0x72, 0x76, 0x69, 0x63,
|
|
0x65, 0x12, 0x51, 0x0a, 0x08, 0x46, 0x65, 0x64, 0x65, 0x72, 0x61, 0x74, 0x65, 0x12, 0x21, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x46, 0x65, 0x64, 0x65, 0x72, 0x61, 0x74, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x1a, 0x22, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x46, 0x65, 0x64, 0x65, 0x72, 0x61, 0x74, 0x65, 0x52, 0x65, 0x73, 0x70,
|
|
0x6f, 0x6e, 0x73, 0x65, 0x12, 0x63, 0x0a, 0x0e, 0x4c, 0x6f, 0x67, 0x69, 0x6e, 0x46, 0x65, 0x64,
|
|
0x65, 0x72, 0x61, 0x74, 0x65, 0x64, 0x12, 0x27, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f,
|
|
0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x4c, 0x6f, 0x67, 0x69, 0x6e, 0x46,
|
|
0x65, 0x64, 0x65, 0x72, 0x61, 0x74, 0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a,
|
|
0x28, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x4c, 0x6f, 0x67, 0x69, 0x6e, 0x46, 0x65, 0x64, 0x65, 0x72, 0x61, 0x74, 0x65,
|
|
0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x42, 0x0a, 0x03, 0x4b, 0x65, 0x79,
|
|
0x12, 0x1c, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x4b, 0x65, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x1d,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x4b, 0x65, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x54, 0x0a,
|
|
0x09, 0x42, 0x65, 0x67, 0x69, 0x6e, 0x41, 0x75, 0x74, 0x68, 0x12, 0x22, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x65,
|
|
0x67, 0x69, 0x6e, 0x41, 0x75, 0x74, 0x68, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x23,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x42, 0x65, 0x67, 0x69, 0x6e, 0x41, 0x75, 0x74, 0x68, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x12, 0x51, 0x0a, 0x08, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74, 0x65, 0x70, 0x12,
|
|
0x21, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74, 0x65, 0x70, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x22, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75,
|
|
0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x4e, 0x65, 0x78, 0x74, 0x53, 0x74, 0x65, 0x70, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x51, 0x0a, 0x08, 0x53, 0x74, 0x65, 0x70, 0x42, 0x61,
|
|
0x63, 0x6b, 0x12, 0x21, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75,
|
|
0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x74, 0x65, 0x70, 0x42, 0x61, 0x63, 0x6b, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x22, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c,
|
|
0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x74, 0x65, 0x70, 0x42, 0x61, 0x63,
|
|
0x6b, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x5c, 0x0a, 0x0b, 0x53, 0x74, 0x72,
|
|
0x65, 0x61, 0x6d, 0x53, 0x74, 0x65, 0x70, 0x73, 0x12, 0x24, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x74, 0x72, 0x65,
|
|
0x61, 0x6d, 0x53, 0x74, 0x65, 0x70, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x25,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x53, 0x74, 0x72, 0x65, 0x61, 0x6d, 0x53, 0x74, 0x65, 0x70, 0x73, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x30, 0x01, 0x12, 0x67, 0x0a, 0x0d, 0x43, 0x68, 0x65, 0x63, 0x6b,
|
|
0x4c, 0x6f, 0x67, 0x67, 0x65, 0x64, 0x49, 0x6e, 0x12, 0x26, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x68, 0x65, 0x63,
|
|
0x6b, 0x4c, 0x6f, 0x67, 0x67, 0x65, 0x64, 0x49, 0x6e, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x1a, 0x27, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x4c, 0x6f, 0x67, 0x67, 0x65, 0x64, 0x49,
|
|
0x6e, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22, 0x05, 0x9a, 0x44, 0x02, 0x08, 0x01,
|
|
0x42, 0xbf, 0x01, 0x0a, 0x14, 0x63, 0x6f, 0x6d, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f,
|
|
0x6c, 0x2e, 0x61, 0x75, 0x74, 0x68, 0x2e, 0x76, 0x31, 0x42, 0x09, 0x41, 0x75, 0x74, 0x68, 0x50,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x50, 0x01, 0x5a, 0x3a, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63,
|
|
0x6f, 0x6d, 0x2f, 0x68, 0x61, 0x72, 0x6d, 0x6f, 0x6e, 0x79, 0x2d, 0x64, 0x65, 0x76, 0x65, 0x6c,
|
|
0x6f, 0x70, 0x6d, 0x65, 0x6e, 0x74, 0x2f, 0x73, 0x68, 0x69, 0x62, 0x73, 0x68, 0x69, 0x62, 0x2f,
|
|
0x67, 0x65, 0x6e, 0x2f, 0x61, 0x75, 0x74, 0x68, 0x2f, 0x76, 0x31, 0x3b, 0x61, 0x75, 0x74, 0x68,
|
|
0x76, 0x31, 0xa2, 0x02, 0x03, 0x50, 0x41, 0x58, 0xaa, 0x02, 0x10, 0x50, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x63, 0x6f, 0x6c, 0x2e, 0x41, 0x75, 0x74, 0x68, 0x2e, 0x56, 0x31, 0xca, 0x02, 0x10, 0x50, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x5c, 0x41, 0x75, 0x74, 0x68, 0x5c, 0x56, 0x31, 0xe2, 0x02,
|
|
0x1c, 0x50, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x5c, 0x41, 0x75, 0x74, 0x68, 0x5c, 0x56,
|
|
0x31, 0x5c, 0x47, 0x50, 0x42, 0x4d, 0x65, 0x74, 0x61, 0x64, 0x61, 0x74, 0x61, 0xea, 0x02, 0x12,
|
|
0x50, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x3a, 0x3a, 0x41, 0x75, 0x74, 0x68, 0x3a, 0x3a,
|
|
0x56, 0x31, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_auth_v1_auth_proto_rawDescOnce sync.Once
|
|
file_auth_v1_auth_proto_rawDescData = file_auth_v1_auth_proto_rawDesc
|
|
)
|
|
|
|
func file_auth_v1_auth_proto_rawDescGZIP() []byte {
|
|
file_auth_v1_auth_proto_rawDescOnce.Do(func() {
|
|
file_auth_v1_auth_proto_rawDescData = protoimpl.X.CompressGZIP(file_auth_v1_auth_proto_rawDescData)
|
|
})
|
|
return file_auth_v1_auth_proto_rawDescData
|
|
}
|
|
|
|
var file_auth_v1_auth_proto_msgTypes = make([]protoimpl.MessageInfo, 26)
|
|
var file_auth_v1_auth_proto_goTypes = []interface{}{
|
|
(*BeginAuthRequest)(nil), // 0: protocol.auth.v1.BeginAuthRequest
|
|
(*BeginAuthResponse)(nil), // 1: protocol.auth.v1.BeginAuthResponse
|
|
(*Session)(nil), // 2: protocol.auth.v1.Session
|
|
(*AuthStep)(nil), // 3: protocol.auth.v1.AuthStep
|
|
(*NextStepRequest)(nil), // 4: protocol.auth.v1.NextStepRequest
|
|
(*NextStepResponse)(nil), // 5: protocol.auth.v1.NextStepResponse
|
|
(*StepBackRequest)(nil), // 6: protocol.auth.v1.StepBackRequest
|
|
(*StepBackResponse)(nil), // 7: protocol.auth.v1.StepBackResponse
|
|
(*StreamStepsRequest)(nil), // 8: protocol.auth.v1.StreamStepsRequest
|
|
(*StreamStepsResponse)(nil), // 9: protocol.auth.v1.StreamStepsResponse
|
|
(*FederateRequest)(nil), // 10: protocol.auth.v1.FederateRequest
|
|
(*FederateResponse)(nil), // 11: protocol.auth.v1.FederateResponse
|
|
(*KeyRequest)(nil), // 12: protocol.auth.v1.KeyRequest
|
|
(*KeyResponse)(nil), // 13: protocol.auth.v1.KeyResponse
|
|
(*LoginFederatedRequest)(nil), // 14: protocol.auth.v1.LoginFederatedRequest
|
|
(*LoginFederatedResponse)(nil), // 15: protocol.auth.v1.LoginFederatedResponse
|
|
(*TokenData)(nil), // 16: protocol.auth.v1.TokenData
|
|
(*CheckLoggedInRequest)(nil), // 17: protocol.auth.v1.CheckLoggedInRequest
|
|
(*CheckLoggedInResponse)(nil), // 18: protocol.auth.v1.CheckLoggedInResponse
|
|
(*AuthStep_Choice)(nil), // 19: protocol.auth.v1.AuthStep.Choice
|
|
(*AuthStep_Form)(nil), // 20: protocol.auth.v1.AuthStep.Form
|
|
(*AuthStep_Waiting)(nil), // 21: protocol.auth.v1.AuthStep.Waiting
|
|
(*AuthStep_Form_FormField)(nil), // 22: protocol.auth.v1.AuthStep.Form.FormField
|
|
(*NextStepRequest_Choice)(nil), // 23: protocol.auth.v1.NextStepRequest.Choice
|
|
(*NextStepRequest_FormFields)(nil), // 24: protocol.auth.v1.NextStepRequest.FormFields
|
|
(*NextStepRequest_Form)(nil), // 25: protocol.auth.v1.NextStepRequest.Form
|
|
(*v1.Token)(nil), // 26: protocol.harmonytypes.v1.Token
|
|
}
|
|
var file_auth_v1_auth_proto_depIdxs = []int32{
|
|
19, // 0: protocol.auth.v1.AuthStep.choice:type_name -> protocol.auth.v1.AuthStep.Choice
|
|
20, // 1: protocol.auth.v1.AuthStep.form:type_name -> protocol.auth.v1.AuthStep.Form
|
|
2, // 2: protocol.auth.v1.AuthStep.session:type_name -> protocol.auth.v1.Session
|
|
21, // 3: protocol.auth.v1.AuthStep.waiting:type_name -> protocol.auth.v1.AuthStep.Waiting
|
|
23, // 4: protocol.auth.v1.NextStepRequest.choice:type_name -> protocol.auth.v1.NextStepRequest.Choice
|
|
25, // 5: protocol.auth.v1.NextStepRequest.form:type_name -> protocol.auth.v1.NextStepRequest.Form
|
|
3, // 6: protocol.auth.v1.NextStepResponse.step:type_name -> protocol.auth.v1.AuthStep
|
|
3, // 7: protocol.auth.v1.StepBackResponse.step:type_name -> protocol.auth.v1.AuthStep
|
|
3, // 8: protocol.auth.v1.StreamStepsResponse.step:type_name -> protocol.auth.v1.AuthStep
|
|
26, // 9: protocol.auth.v1.FederateResponse.token:type_name -> protocol.harmonytypes.v1.Token
|
|
26, // 10: protocol.auth.v1.LoginFederatedRequest.auth_token:type_name -> protocol.harmonytypes.v1.Token
|
|
2, // 11: protocol.auth.v1.LoginFederatedResponse.session:type_name -> protocol.auth.v1.Session
|
|
22, // 12: protocol.auth.v1.AuthStep.Form.fields:type_name -> protocol.auth.v1.AuthStep.Form.FormField
|
|
24, // 13: protocol.auth.v1.NextStepRequest.Form.fields:type_name -> protocol.auth.v1.NextStepRequest.FormFields
|
|
10, // 14: protocol.auth.v1.AuthService.Federate:input_type -> protocol.auth.v1.FederateRequest
|
|
14, // 15: protocol.auth.v1.AuthService.LoginFederated:input_type -> protocol.auth.v1.LoginFederatedRequest
|
|
12, // 16: protocol.auth.v1.AuthService.Key:input_type -> protocol.auth.v1.KeyRequest
|
|
0, // 17: protocol.auth.v1.AuthService.BeginAuth:input_type -> protocol.auth.v1.BeginAuthRequest
|
|
4, // 18: protocol.auth.v1.AuthService.NextStep:input_type -> protocol.auth.v1.NextStepRequest
|
|
6, // 19: protocol.auth.v1.AuthService.StepBack:input_type -> protocol.auth.v1.StepBackRequest
|
|
8, // 20: protocol.auth.v1.AuthService.StreamSteps:input_type -> protocol.auth.v1.StreamStepsRequest
|
|
17, // 21: protocol.auth.v1.AuthService.CheckLoggedIn:input_type -> protocol.auth.v1.CheckLoggedInRequest
|
|
11, // 22: protocol.auth.v1.AuthService.Federate:output_type -> protocol.auth.v1.FederateResponse
|
|
15, // 23: protocol.auth.v1.AuthService.LoginFederated:output_type -> protocol.auth.v1.LoginFederatedResponse
|
|
13, // 24: protocol.auth.v1.AuthService.Key:output_type -> protocol.auth.v1.KeyResponse
|
|
1, // 25: protocol.auth.v1.AuthService.BeginAuth:output_type -> protocol.auth.v1.BeginAuthResponse
|
|
5, // 26: protocol.auth.v1.AuthService.NextStep:output_type -> protocol.auth.v1.NextStepResponse
|
|
7, // 27: protocol.auth.v1.AuthService.StepBack:output_type -> protocol.auth.v1.StepBackResponse
|
|
9, // 28: protocol.auth.v1.AuthService.StreamSteps:output_type -> protocol.auth.v1.StreamStepsResponse
|
|
18, // 29: protocol.auth.v1.AuthService.CheckLoggedIn:output_type -> protocol.auth.v1.CheckLoggedInResponse
|
|
22, // [22:30] is the sub-list for method output_type
|
|
14, // [14:22] is the sub-list for method input_type
|
|
14, // [14:14] is the sub-list for extension type_name
|
|
14, // [14:14] is the sub-list for extension extendee
|
|
0, // [0:14] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_auth_v1_auth_proto_init() }
|
|
func file_auth_v1_auth_proto_init() {
|
|
if File_auth_v1_auth_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_auth_v1_auth_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BeginAuthRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BeginAuthResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Session); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*AuthStep); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NextStepRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NextStepResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StepBackRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StepBackResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StreamStepsRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[9].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StreamStepsResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[10].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*FederateRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[11].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*FederateResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[12].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*KeyRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[13].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*KeyResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[14].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*LoginFederatedRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[15].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*LoginFederatedResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[16].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*TokenData); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[17].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*CheckLoggedInRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[18].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*CheckLoggedInResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[19].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*AuthStep_Choice); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[20].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*AuthStep_Form); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[21].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*AuthStep_Waiting); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[22].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*AuthStep_Form_FormField); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[23].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NextStepRequest_Choice); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[24].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NextStepRequest_FormFields); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[25].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NextStepRequest_Form); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[3].OneofWrappers = []interface{}{
|
|
(*AuthStep_Choice_)(nil),
|
|
(*AuthStep_Form_)(nil),
|
|
(*AuthStep_Session)(nil),
|
|
(*AuthStep_Waiting_)(nil),
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[4].OneofWrappers = []interface{}{
|
|
(*NextStepRequest_Choice_)(nil),
|
|
(*NextStepRequest_Form_)(nil),
|
|
}
|
|
file_auth_v1_auth_proto_msgTypes[16].OneofWrappers = []interface{}{}
|
|
file_auth_v1_auth_proto_msgTypes[24].OneofWrappers = []interface{}{
|
|
(*NextStepRequest_FormFields_Bytes)(nil),
|
|
(*NextStepRequest_FormFields_String_)(nil),
|
|
(*NextStepRequest_FormFields_Number)(nil),
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_auth_v1_auth_proto_rawDesc,
|
|
NumEnums: 0,
|
|
NumMessages: 26,
|
|
NumExtensions: 0,
|
|
NumServices: 1,
|
|
},
|
|
GoTypes: file_auth_v1_auth_proto_goTypes,
|
|
DependencyIndexes: file_auth_v1_auth_proto_depIdxs,
|
|
MessageInfos: file_auth_v1_auth_proto_msgTypes,
|
|
}.Build()
|
|
File_auth_v1_auth_proto = out.File
|
|
file_auth_v1_auth_proto_rawDesc = nil
|
|
file_auth_v1_auth_proto_goTypes = nil
|
|
file_auth_v1_auth_proto_depIdxs = nil
|
|
}
|