mirror of
https://github.com/cwinfo/matterbridge.git
synced 2024-11-10 20:20:27 +00:00
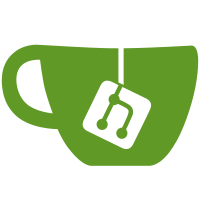
* Add vk bridge * Vk bridge attachments * Vk bridge forwarded messages * Vk bridge sample config and code cleanup * Vk bridge add vendor * Vk bridge message edit * Vk bridge: fix fetching names of other bots * Vk bridge: code cleanup * Vk bridge: fix shadows declaration * Vk bridge: remove UseFileURL
150 lines
4.3 KiB
Go
150 lines
4.3 KiB
Go
package api // import "github.com/SevereCloud/vksdk/v2/api"
|
|
|
|
import (
|
|
"github.com/SevereCloud/vksdk/v2/object"
|
|
)
|
|
|
|
// AppsDeleteAppRequests deletes all request notifications from the current app.
|
|
//
|
|
// https://vk.com/dev/apps.deleteAppRequests
|
|
func (vk *VK) AppsDeleteAppRequests(params Params) (response int, err error) {
|
|
err = vk.RequestUnmarshal("apps.deleteAppRequests", &response, params)
|
|
return
|
|
}
|
|
|
|
// AppsGetResponse struct.
|
|
type AppsGetResponse struct {
|
|
Count int `json:"count"`
|
|
Items []object.AppsApp `json:"items"`
|
|
object.ExtendedResponse
|
|
}
|
|
|
|
// AppsGet returns applications data.
|
|
//
|
|
// https://vk.com/dev/apps.get
|
|
func (vk *VK) AppsGet(params Params) (response AppsGetResponse, err error) {
|
|
err = vk.RequestUnmarshal("apps.get", &response, params)
|
|
return
|
|
}
|
|
|
|
// AppsGetCatalogResponse struct.
|
|
type AppsGetCatalogResponse struct {
|
|
Count int `json:"count"`
|
|
Items []object.AppsApp `json:"items"`
|
|
object.ExtendedResponse
|
|
}
|
|
|
|
// AppsGetCatalog returns a list of applications (apps) available to users in the App Catalog.
|
|
//
|
|
// https://vk.com/dev/apps.getCatalog
|
|
func (vk *VK) AppsGetCatalog(params Params) (response AppsGetCatalogResponse, err error) {
|
|
err = vk.RequestUnmarshal("apps.getCatalog", &response, params)
|
|
return
|
|
}
|
|
|
|
// AppsGetFriendsListResponse struct.
|
|
type AppsGetFriendsListResponse struct {
|
|
Count int `json:"count"`
|
|
Items []int `json:"items"`
|
|
}
|
|
|
|
// AppsGetFriendsList creates friends list for requests and invites in current app.
|
|
//
|
|
// extended=0
|
|
//
|
|
// https://vk.com/dev/apps.getFriendsList
|
|
func (vk *VK) AppsGetFriendsList(params Params) (response AppsGetFriendsListResponse, err error) {
|
|
err = vk.RequestUnmarshal("apps.getFriendsList", &response, params, Params{"extended": false})
|
|
|
|
return
|
|
}
|
|
|
|
// AppsGetFriendsListExtendedResponse struct.
|
|
type AppsGetFriendsListExtendedResponse struct {
|
|
Count int `json:"count"`
|
|
Items []object.UsersUser `json:"items"`
|
|
}
|
|
|
|
// AppsGetFriendsListExtended creates friends list for requests and invites in current app.
|
|
//
|
|
// extended=1
|
|
//
|
|
// https://vk.com/dev/apps.getFriendsList
|
|
func (vk *VK) AppsGetFriendsListExtended(params Params) (response AppsGetFriendsListExtendedResponse, err error) {
|
|
err = vk.RequestUnmarshal("apps.getFriendsList", &response, params, Params{"extended": true})
|
|
|
|
return
|
|
}
|
|
|
|
// AppsGetLeaderboardResponse struct.
|
|
type AppsGetLeaderboardResponse struct {
|
|
Count int `json:"count"`
|
|
Items []object.AppsLeaderboard `json:"items"`
|
|
}
|
|
|
|
// AppsGetLeaderboard returns players rating in the game.
|
|
//
|
|
// extended=0
|
|
//
|
|
// https://vk.com/dev/apps.getLeaderboard
|
|
func (vk *VK) AppsGetLeaderboard(params Params) (response AppsGetLeaderboardResponse, err error) {
|
|
err = vk.RequestUnmarshal("apps.getLeaderboard", &response, params, Params{"extended": false})
|
|
|
|
return
|
|
}
|
|
|
|
// AppsGetLeaderboardExtendedResponse struct.
|
|
type AppsGetLeaderboardExtendedResponse struct {
|
|
Count int `json:"count"`
|
|
Items []struct {
|
|
Score int `json:"score"`
|
|
UserID int `json:"user_id"`
|
|
} `json:"items"`
|
|
Profiles []object.UsersUser `json:"profiles"`
|
|
}
|
|
|
|
// AppsGetLeaderboardExtended returns players rating in the game.
|
|
//
|
|
// extended=1
|
|
//
|
|
// https://vk.com/dev/apps.getLeaderboard
|
|
func (vk *VK) AppsGetLeaderboardExtended(params Params) (response AppsGetLeaderboardExtendedResponse, err error) {
|
|
err = vk.RequestUnmarshal("apps.getLeaderboard", &response, params, Params{"extended": true})
|
|
|
|
return
|
|
}
|
|
|
|
// AppsGetScopesResponse struct.
|
|
type AppsGetScopesResponse struct {
|
|
Count int `json:"count"`
|
|
Items []object.AppsScope `json:"items"`
|
|
}
|
|
|
|
// AppsGetScopes ...
|
|
//
|
|
// TODO: write docs.
|
|
//
|
|
// https://vk.com/dev/apps.getScopes
|
|
func (vk *VK) AppsGetScopes(params Params) (response AppsGetScopesResponse, err error) {
|
|
err = vk.RequestUnmarshal("apps.getScopes", &response, params)
|
|
return
|
|
}
|
|
|
|
// AppsGetScore returns user score in app.
|
|
//
|
|
// NOTE: vk wtf!?
|
|
//
|
|
// https://vk.com/dev/apps.getScore
|
|
func (vk *VK) AppsGetScore(params Params) (response string, err error) {
|
|
err = vk.RequestUnmarshal("apps.getScore", &response, params)
|
|
return
|
|
}
|
|
|
|
// AppsSendRequest sends a request to another user in an app that uses VK authorization.
|
|
//
|
|
// https://vk.com/dev/apps.sendRequest
|
|
func (vk *VK) AppsSendRequest(params Params) (response int, err error) {
|
|
err = vk.RequestUnmarshal("apps.sendRequest", &response, params)
|
|
return
|
|
}
|