mirror of
https://github.com/cwinfo/matterbridge.git
synced 2024-11-10 12:10:26 +00:00
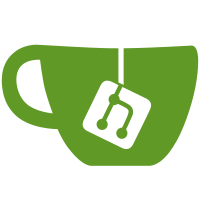
TengoModifyMessage allows you to specify the location of a tengo (https://github.com/d5/tengo/) script. This script will receive every incoming message and can be used to modify the Username and the Text of that message. The script will have the following global variables: to modify: msgUsername and msgText to read: msgChannel and msgAccount The script is reloaded on every message, so you can modify the script on the fly. Example script can be found in https://github.com/42wim/matterbridge/tree/master/gateway/bench.tengo and https://github.com/42wim/matterbridge/tree/master/contrib/example.tengo The example below will check if the text contains blah and if so, it'll replace the text and the username of that message. text := import("text") if text.re_match("blah",msgText) { msgText="replaced by this" msgUsername="fakeuser" } More information about tengo on: https://github.com/d5/tengo/blob/master/docs/tutorial.md and https://github.com/d5/tengo/blob/master/docs/stdlib.md
114 lines
2.4 KiB
Go
114 lines
2.4 KiB
Go
package script
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/d5/tengo/compiler"
|
|
"github.com/d5/tengo/objects"
|
|
"github.com/d5/tengo/runtime"
|
|
)
|
|
|
|
// Compiled is a compiled instance of the user script.
|
|
// Use Script.Compile() to create Compiled object.
|
|
type Compiled struct {
|
|
symbolTable *compiler.SymbolTable
|
|
machine *runtime.VM
|
|
}
|
|
|
|
// Run executes the compiled script in the virtual machine.
|
|
func (c *Compiled) Run() error {
|
|
return c.machine.Run()
|
|
}
|
|
|
|
// RunContext is like Run but includes a context.
|
|
func (c *Compiled) RunContext(ctx context.Context) (err error) {
|
|
ch := make(chan error, 1)
|
|
|
|
go func() {
|
|
ch <- c.machine.Run()
|
|
}()
|
|
|
|
select {
|
|
case <-ctx.Done():
|
|
c.machine.Abort()
|
|
<-ch
|
|
err = ctx.Err()
|
|
case err = <-ch:
|
|
}
|
|
|
|
return
|
|
}
|
|
|
|
// IsDefined returns true if the variable name is defined (has value) before or after the execution.
|
|
func (c *Compiled) IsDefined(name string) bool {
|
|
symbol, _, ok := c.symbolTable.Resolve(name)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
v := c.machine.Globals()[symbol.Index]
|
|
if v == nil {
|
|
return false
|
|
}
|
|
|
|
return *v != objects.UndefinedValue
|
|
}
|
|
|
|
// Get returns a variable identified by the name.
|
|
func (c *Compiled) Get(name string) *Variable {
|
|
value := &objects.UndefinedValue
|
|
|
|
symbol, _, ok := c.symbolTable.Resolve(name)
|
|
if ok && symbol.Scope == compiler.ScopeGlobal {
|
|
value = c.machine.Globals()[symbol.Index]
|
|
if value == nil {
|
|
value = &objects.UndefinedValue
|
|
}
|
|
}
|
|
|
|
return &Variable{
|
|
name: name,
|
|
value: value,
|
|
}
|
|
}
|
|
|
|
// GetAll returns all the variables that are defined by the compiled script.
|
|
func (c *Compiled) GetAll() []*Variable {
|
|
var vars []*Variable
|
|
for _, name := range c.symbolTable.Names() {
|
|
symbol, _, ok := c.symbolTable.Resolve(name)
|
|
if ok && symbol.Scope == compiler.ScopeGlobal {
|
|
value := c.machine.Globals()[symbol.Index]
|
|
if value == nil {
|
|
value = &objects.UndefinedValue
|
|
}
|
|
|
|
vars = append(vars, &Variable{
|
|
name: name,
|
|
value: value,
|
|
})
|
|
}
|
|
}
|
|
|
|
return vars
|
|
}
|
|
|
|
// Set replaces the value of a global variable identified by the name.
|
|
// An error will be returned if the name was not defined during compilation.
|
|
func (c *Compiled) Set(name string, value interface{}) error {
|
|
obj, err := objects.FromInterface(value)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
symbol, _, ok := c.symbolTable.Resolve(name)
|
|
if !ok || symbol.Scope != compiler.ScopeGlobal {
|
|
return fmt.Errorf("'%s' is not defined", name)
|
|
}
|
|
|
|
c.machine.Globals()[symbol.Index] = &obj
|
|
|
|
return nil
|
|
}
|